NASA Power of 10 - Objective-C, Swift & SwiftUI Approach
Posted in Engineering on IOS Engineering
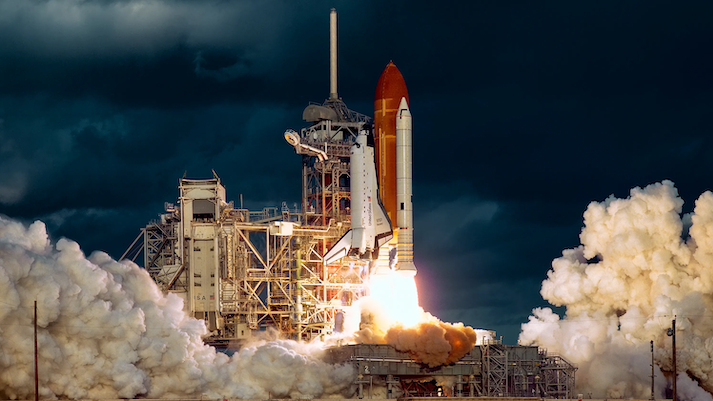
The Power of 10 rules were created in 2006 by Gerard J. Holzmann of the NASA/JPL Laboratory of Reliable Software
The most serious software development projects are governed by a set of coding guidelines that aim to be the backbone of how exactly code should be submitted inorder to guarantee utmost success.
Rule 1: Restrict Use of Dynamic Memory Allocation
Dynamic memory allocation (e.g., malloc
, free
, new
, delete
) can lead to memory fragmentation, leaks, and unpredictable behavior. iOS apps should favor static or stack-based allocation and use ARC (Automatic Reference Counting) in Swift.
Best Practices in iOS
- Use value types (
structs
) in Swift instead of reference types (classes
) where possible. - Avoid excessive use of
NSMutableArray
,NSMutableDictionary
in Objective-C; prefer immutable collections. - Utilize Swift’s ARC to manage memory efficiently.
- Avoid allocating memory in loops or frequently called functions.
Swift
struct User {
let id: Int
let name: String
}
// Prefer value types (struct) to avoid unnecessary heap allocation.
let user = User(id: 1, name: "Alice")
Good iterative approach to Swift
Objective-C
@interface User : NSObject
@property (nonatomic, assign) int userId;
@property (nonatomic, strong) NSString *name;
@end
@implementation User
@end
User *user = [[User alloc] init];
user.userId = 1;
user.name = @"Alice";
SwiftUI
struct ContentView: View {
let users = ["Alice", "Bob", "Charlie"] // Avoids dynamic allocation in loops
var body: some View {
List(users, id: \ .self) { user in
Text(user)
}
}
}