AsyncAwait - BackPressure Management
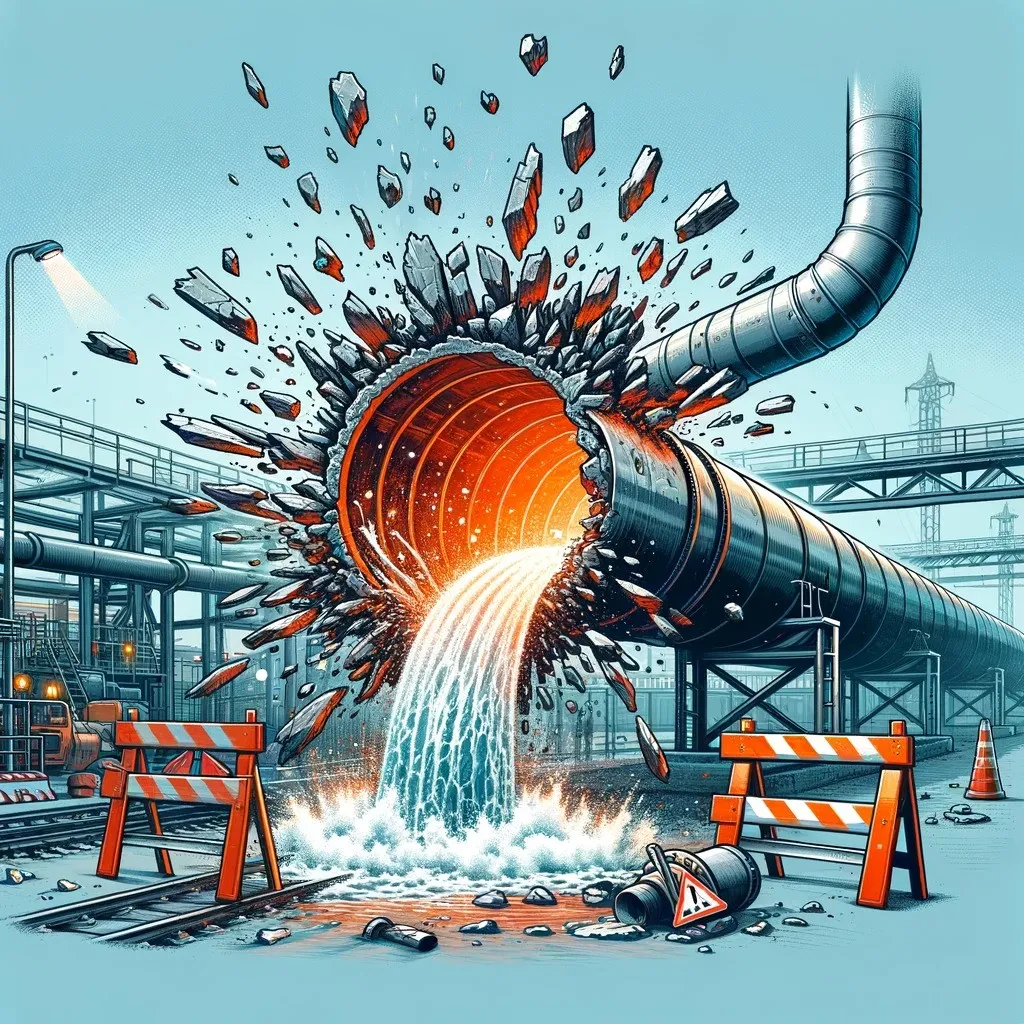
Advanced Backpressure Support for AsyncStream in Swift.
Overview
The Swift Evolution Proposal SE-0406 introduces enhancements to AsyncStream
and AsyncThrowingStream
by adding backpressure support. This feature is crucial for efficiently bridging synchronous and asynchronous data streams while ensuring optimal resource utilization in iOS development.
Motivation
Current implementations of AsyncStream
and AsyncThrowingStream
provide an essential means to convert delegate-based or callback-based APIs into structured AsyncSequence
types. However, they fall short in handling:
- Backpressure: Lacks explicit flow control for signaling when to pause and resume production.
- Consumer Management: Does not properly differentiate between unicast and multicast behavior.
- Termination Handling: Does not clearly define behavior when consumers or producers terminate unexpectedly.
The proposal aims to refine these behaviors, making AsyncStream
more suitable for real-world iOS applications handling network requests, data streams, and UI-driven async operations.
Key Enhancements
1. Backpressure Management
A new backpressure strategy is introduced, enabling precise control over data flow between the producer and consumer:
let (stream, source) = AsyncStream.makeStream(
of: Int.self,
backpressureStrategy: .watermark(low: 2, high: 4)
)
- Uses a watermark-based system where producers are paused when the buffer reaches a high threshold and resumed when it drops below a low threshold.
- Supports both immediate production (
produceMore
) and callback-based production resumption (enqueueCallback
).
2. Enhanced Consumer Handling
- Introduces strict unicast behavior, ensuring that only one consumer can iterate at a time.
- Prevents
fatalError
scenarios when multiple iterators are created.
3. Improved Termination Handling
- Consumers can now gracefully terminate via
source.finish()
. - Producers are automatically notified when consumers cancel the stream or reach completion.
4. New Writing APIs
The API introduces multiple ways to write data to the stream, providing flexibility for different use cases:
a) Immediate Write with Backpressure Handling
let writeResult = try source.write(contentsOf: sequence)
- If the buffer is full, it returns
.enqueueCallback(token)
, allowing the producer to be notified when to resume.
b) Asynchronous Write (Suspends Until Consumption)
try await source.write(contentsOf: sequence)
- Ensures that production resumes only when backpressure allows it.
c) Write with Explicit Callback for Resumption
try source.write(contentsOf: sequence, onProduceMore: { result in
switch result {
case .success:
// Resume production
case .failure(let error):
// Handle termination
}
})
5. Producer Termination Notifications
Producers can now be explicitly notified when consumers stop consuming, preventing resource leaks.
source.onTermination = {
print("Consumer terminated, cleaning up producer...")
}
Applications in iOS Development
1. Real-time Data Streaming (e.g., WebSockets, Live Feeds)
Backpressure ensures that high-frequency data streams do not overwhelm memory by controlling the data flow efficiently.
Task {
let (stream, source) = AsyncStream.makeStream(of: Data.self, backpressureStrategy: .watermark(low: 5, high: 10))
for await data in stream {
processData(data)
}
source.finish()
}
2. Network Request Handling
Efficiently bridges URLSession-based data tasks into async sequences without excessive buffering.
let (stream, source) = AsyncStream.makeStream(of: Data.self, backpressureStrategy: .watermark(low: 2, high: 4))
fetchData { chunk in
try? source.write(chunk)
}
3. Async UI Updates (e.g., Infinite Scrolling, Data Pagination)
Ensures that UI remains responsive by pacing data retrieval based on user scroll behavior.
func loadMoreData() async {
let (stream, source) = AsyncStream.makeStream(of: Item.self, backpressureStrategy: .watermark(low: 5, high: 15))
Task {
for await item in stream {
updateUI(with: item)
}
}
try await source.write(contentsOf: fetchNextBatch())
}
Future Considerations
- Adaptive Backpressure Strategies: Dynamically adjust thresholds based on system load.
- Size-dependent Strategies: Consider memory footprint instead of just element count.
- Integration with Swift Concurrency: Extend
AsyncStream
to support structured concurrency patterns.
Conclusion
SE-0406 introduces a robust and efficient mechanism to integrate backpressure-aware async streams into Swift. These improvements provide significant advantages in networking, data streaming, and UI-driven async workflows for iOS applications, ensuring optimal performance and resource management.